Lottie is an animation library by AirBnb that makes it super simple to add animation in any native app – web and mobile. It helps bridge the gap from designers designing an animation to developers implementing it in the app. In this post, we will see how we can add animations to enhance our Xamarin.Forms app experience with minimal effort (the process is similar for Native apps as well).
Thanks to Martijn van Dijk, we can now simply use his NuGet Package to bring animations to our Xamarin.Forms app in a jiffy.
Animations
Custom Animation
First step is to create an animation using After Effects. Once the animation is created and ready to be tested, we need to convert it to a json
format to be embedded in the app. To do this, install Bodymovin plugin.
“Bodymovin is a plugin for After Effects that can export animation in json data format for Lottie to use.”
airbnb.design/lottie
This part is usually on the UI/UX designers and engineers to take of. Once they have their json file ready, they can preview at LottieFiles.
Community Animation
I cannot stress the fact how much I am impressed by community contributions to Lottie animations ready to be consumed. Make sure to checkout LottieFiles community section to find some great animations that you can use right away.
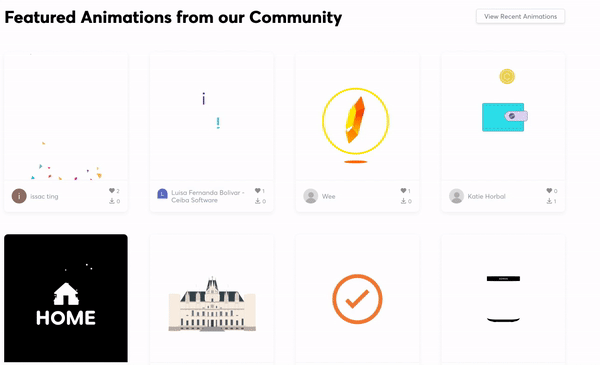
The check animation I am using comes from LottieFiles as well. Once you decide on the animation you want, simply download the json file.
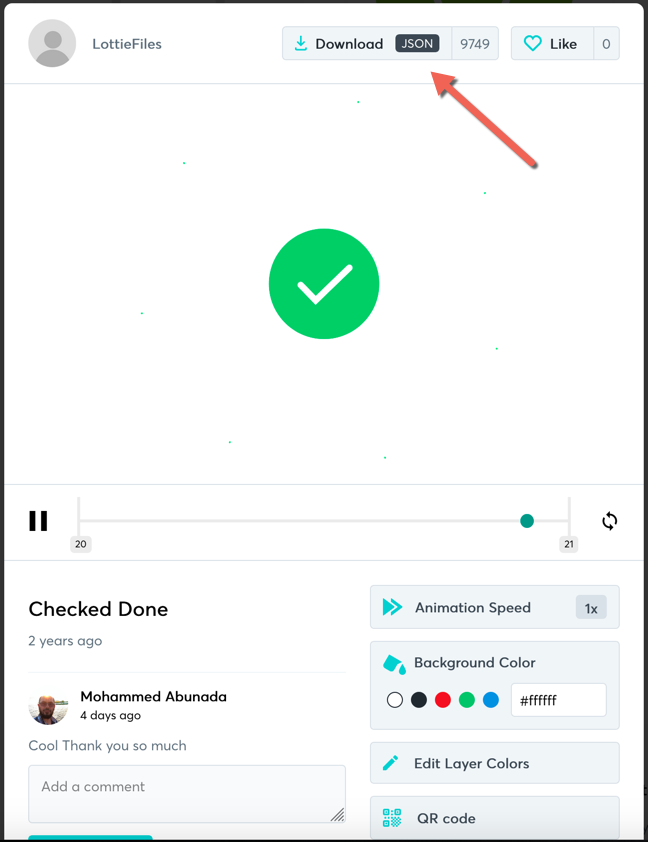
Import In Xamarin.Forms Solution
In our Xamarin.Forms app, The imported json
goes under Assets
folder for Android with Build Action set to AndroidAsset
, and can be under Resources
folder for iOS with Build Action set to BundledResource
.
Add Animation
Now that we have the animation assets ready to go, let’s get started with the UI. Add the Com.Airbnb.Xamarin.Forms.Lottie NuGet package by Martijn van Dijk to our shared and platform projects.
At this point, you can follow a generic route to show animations in your app as shown in this blog.
xmlns:lottie="clr-namespace:Lottie.Forms;assembly=Lottie.Forms"
...
<lottie:AnimationView
Animation="LottieLogo1.json"
Loop="True"
AutoPlay="True" />
However, if you want to integrate these animations seamlessly in your app, keep reading.
Integrating Animations In Controls (Optional but useful)
It is sufficient to simply add an AnimationView
on any page and have animations play. But if you are like me who likes to keep things simply by abstracting libraries away in case we have to change the implementation/library/package in the future. For example, many of us swapped out several plugins/libraries with one Xamarin.Essentials. This practice makes sure that if and when you change the library, you only have to update your implementation/class/control/etc. instead of going all over the app to make changes.
Anyway, let’s take a simple checklist app.
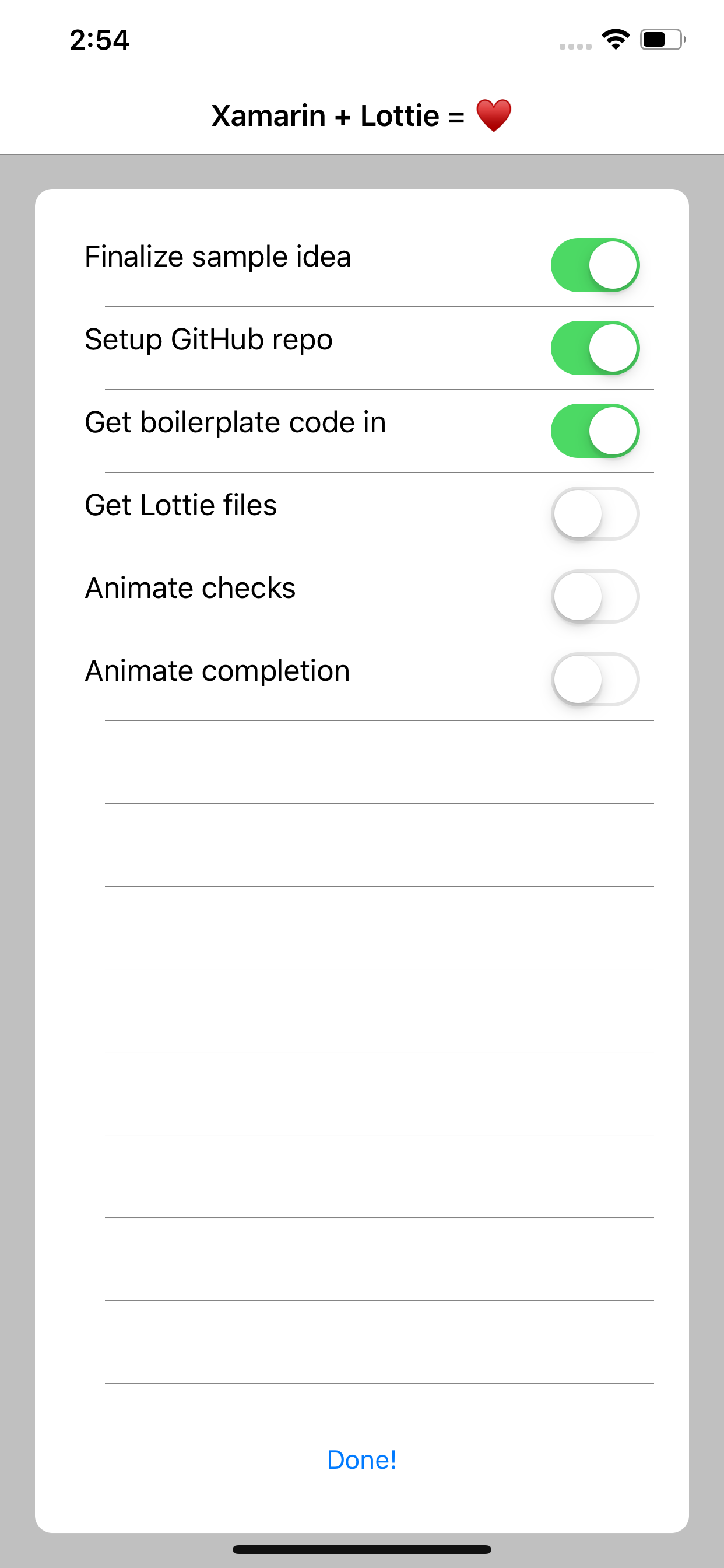
What we want to do is to enhance the UI to make it stand out. We will start by creating a simple custom control FancyCheckbox
that extends the AnimationView
in the Lottie.Forms
namespace.
Remember, it’s all about the
AnimationView
. The animation runs inside that view
Fancy Checkbox
public class FancyCheckbox : AnimationView {}
Inside this custom control, we will introduce a single IsChecked
bindable property. We can then control the animation based on this property.
public static BindableProperty IsCheckedProperty = BindableProperty.Create(nameof(IsChecked), typeof(bool), typeof(FancyCheckbox), defaultBindingMode:BindingMode.TwoWay, propertyChanged:IsCheckedChanged);
public bool IsChecked
{
get { return (bool) GetValue(IsCheckedProperty); }
set { SetValue(IsCheckedProperty, value); }
}
static void IsCheckedChanged(BindableObject bindable, object oldValue, object newValue)
{
if (!(bindable is FancyCheckbox cb))
return;
if ((bool)newValue)
cb.Play();
else
cb.Reset();
}
We can now instantiate this control with the animation file we need and handle the OnClick()
event.
public FancyCheckbox()
{
Animation = "check.json";
OnClick += Checkbox_OnClick;
}
void Checkbox_OnClick(object sender, EventArgs e)
{
IsChecked = !IsChecked;
}
Now we are ready to use this custom control in our views without having to worry about Lottie and it’s namespaces everywhere. Like this,
<local:FancyCheckbox IsChecked="{Binding IsComplete}" />
Result,

Note: You can still bring in
AnimationView
as needed without always wrapping it in a control. For example, you may have a custom popup with animation in it, you don’t need a custom control for it, simply add the animation and play it on appearance.
Use Cases
Here are some of the use cases that can benefit from these animations,
- Splash screen animation
- Selection controls (checkbox, multi-select, etc.)
- Confirmation checks or warnings
- Transitions
- Interactions with ListView (add, remove items)
- and many more
That is it. Simple steps to go from generic out-of-box experience to polished UI and enhanced user experience. Let me know in the comments how you are using or planning to use Lottie in your Xamarin.Forms app.
Enjoy!
Sample code: https://github.com/Intelliabb/XamarinLottie
Hi, in android when uncheck, the animation is not reset, the green color still exist. Any advise?
Make sure the control is reset. I don’t see that on my end, but I imagine that’s what’s going on. Let me know if resetting helps.